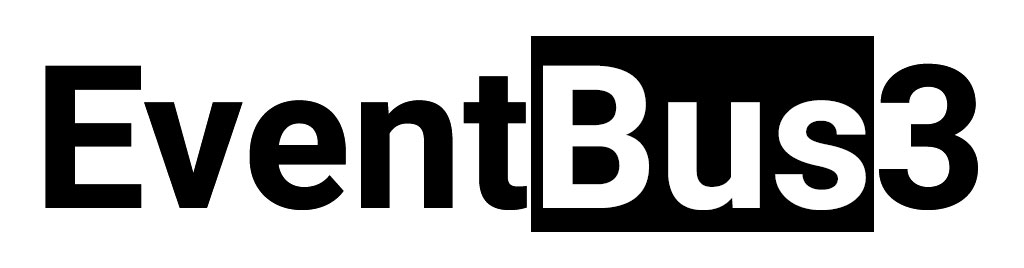
Implementing publisher/subscriber pattern using EventBus
Publisher/subscriber pattern is widely used in Android applications. It is mostly used in Activity-Fragment communication, Services and AsyncTasks. In this pattern, a subscriber waits a message from publisher when something happens. This message can be anything.
In this article, I will introduce you EventBus library. It is a very useful implementation of pub/sub pattern.
Publish / Subscribe pattern
Publish / subscribe pattern provides s messaging system between publishers and subscribers. Subscribers registers to publishers and start waiting for a certain event occurs.
When this event occurs, publisher notyfies all of its subscribers via the event bus.
EventBus library
EventBus is developed by Greenrobot team. You can add it to your project by
compile 'org.greenrobot:eventbus:3.0.0'
From now on, we can use EventBus! Ok let’s start.
First of all, we have to register our activity (or class you are using) to event bus.
@Override
public void onStart() {
super.onStart();
EventBus.getDefault().register(this);
}
@Override
public void onStop() {
EventBus.getDefault().unregister(this);
super.onStop();
}
We said that publishers send messages to subscribers when a certain event occurs. So lets define a message.
public class Message{
private final String message;
public Message(String message) {
this.message = message;
}
public String getMessage() {
return message;
}
}
When an event occurs, a Message object will be produced and sent it to the subscriber. Thanks to the EventBus, the only thing we have to do is adding @Subscribe annotation to our method. Note that, here threadMode is optional.
@Subscribe(threadMode = ThreadMode.MAIN)
public void onMessage(Message event){
myTextView.setText(event.getMessage());
}
Now that we subscribe to Message event, we can get events when they are posted by publisher. Posting events are also easy.
EventBus.getDefault().post(new Message("Message event is occured"));
Sticky events
When an activity is waiting an event, it can be paused by another activity. In this scenario, activity won’t be able to get event even if it resumes again. For overcoming this problem, sticky events can be used.
Sticky events are posted by publisher and lives until they are consumed by a subscriber. In this way, if an event is posted when subscriber is at pause state, subscriber can get the event even once it resumes again. Sticky events is posted as follows:
EventBus.getDefault().postSticky(new MessageEvent("Sticky message"));
And they are consumed as follows:
@Subscribe(threadMode = ThreadMode.MAIN, sticky = true)
public void onMessage(Message event) {
myTextView.setText(event.getMessage());
}
Conclusion
In this post, I tried to explain publish/subscribe pattern. This pattern is very useful for communicating two classes. Instead of building this pattern, we can use EventBus library. I introduced EventBus and gave some usage examples. More documentation can be found at Greenrobot.