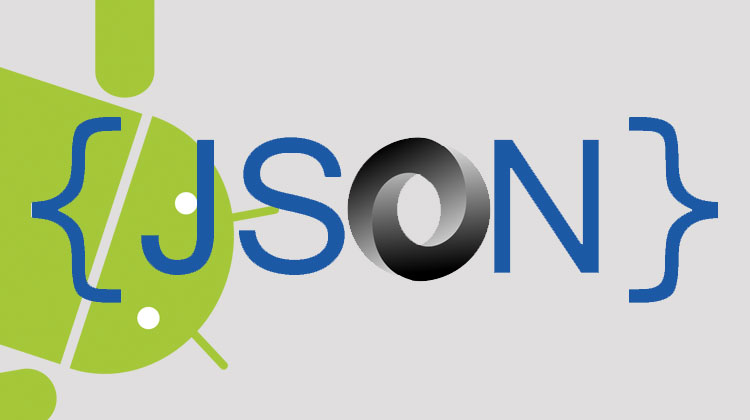
Easily generate JSON model from JSON string using GsonFormat
In this post, I will explain a very useful tool, GsonFormat, for Android Studio, which can convert a JSON string to a Java class.
Almost every software needs to communicate with a remote computer and get/post info between them. When exchanging data, the data can be transformed into a text. JSON is text, and we can convert any JavaScript object into JSON, and send JSON to the remote computer (I will call it “server” from now on). We can also convert any JSON received from the server into JavaScript objects. This way we can work with the data as JavaScript objects, with no complicated parsing and translations.
Creating the classes from JSON strings can be really annoying especially if the JSON data includes nested arrays and objects. Instead of trying to do this, we can use GsonFormat and it automatically creates the Java classes for us.
Installing GsonFormat
GsonFormat is a plugin that can be easily installed to Android Studio.
- Using IDE built-in plugin system on Windows:
- File > Settings > Plugins > Browse repositories… > Search for “GsonFormat” > Install Plugin
- Using IDE built-in plugin system on MacOs:
- Preferences > Settings > Plugins > Browse repositories… > Search for “GsonFormat” > Install Plugin
- Manually:
- Download the latest release and install it manually using Preferences > Plugins > Install plugin from disk…
- From official jetbrains store from download
Don’t forget to restart IDE.
Usage
I used the below JSON string for example.
{"menu": {
"id": "file",
"value": "File",
"popup": {
"menuitem": [
{"value": "New", "onclick": "CreateNewDoc()"},
{"value": "Open", "onclick": "OpenDoc()"},
{"value": "Close", "onclick": "CloseDoc()"}
]
}
}}
After installation, just go to Code > Generate > GsonFormat
A text panel will be prompt to us. Paste your JSON string here and press Format button. And lastly, press OK button.
When you pressed OK, it will show you the variables and their types. You can change them from this window if you want. After that, you can again press OK button and finish.
Thats all! It creates the class variables, methods and everything itself.
And this is the result:
public static class MenuBean {
private String id;
private String value;
private PopupBean popup;
public String getİd() { return id; }
public void setİd(String id) { this.id = id; }
public String getValue() { return value; }
public void setValue(String value) { this.value = value; }
public PopupBean getPopup() { return popup; }
public void setPopup(PopupBean popup) { this.popup = popup; }
public static class PopupBean {
private List<MenuitemBean> menuitem;
public List<MenuitemBean> getMenuitem() { return menuitem; }
public void setMenuitem(List<MenuitemBean> menuitem) { this.menuitem = menuitem; }
public static class MenuitemBean {
private String value;
private String onclick;
public String getValue() { return value; }
public void setValue(String value) { this.value = value; }
public String getOnclick() { return onclick; }
public void setOnclick(String onclick) { this.onclick = onclick; }
}
}
}
Conclusion
Converting JSON strings to JSON classes is a time consuming task. Creating the appropriate classes can be annoying. With GsonFormat, we don’t have to do it anymore. Every Android developer should use this awesome tool.