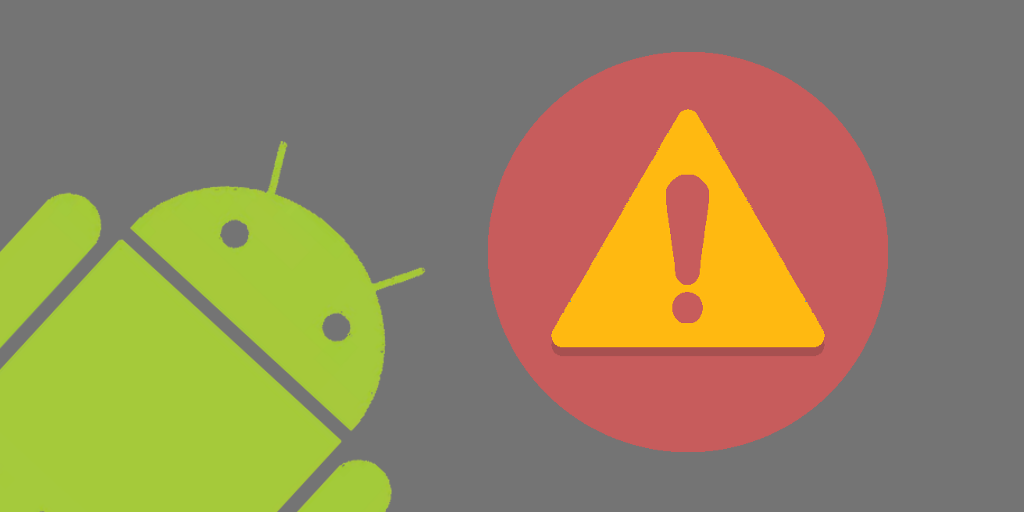
Create awesome warnings using Noty
In mobile apps, we would like to show little warnings/dialogs/notification when an important thing happens. This important thing can be a download complete notification, a message alert or an update required warning. In this way we inform the user and they feel more confortable. For this purpose here is Noty, an open source library for creating animated custom warnings/dialogs.
Installation
Adding Noty to your application is super easy. Just add the line below to your app’s gradle file and click sync the project
compile 'com.emredavarci:noty:1.0.3'
Usage
Noty is providing us to customize almost everything. You can find the full list of the customization methods here.
Now lets look at an example given at the Noty github page. You can also find all example codes here.
The simplest usage of Noty is this:
/*
* rl is the layout we are working on.
*/
RelativeLayout rl = (RelativeLayout) findViewById(R.id.myLayout) ;
Noty.init(YourActivity.this
,"Your warning message"
,yourLayout, Noty.WarningStyle.SIMPLE)
.show();
Note that you need to get reference of the layout that you want to use Noty.
This example is only including a message. but we can also add an action button too. At below there is an example of that.
Noty.init(YourActivity.this, "Your warning message", yourLayout,
Noty.WarningStyle.ACTION)
.setActionText("OK").show();
Ok, lets go deeper and add some customization to it.
Noty.init(YourActivity.this, "Your warning message", yourLayout,
Noty.WarningStyle.ACTION)
.setActionText("OK")
.setWarningBoxBgColor("#ff5c33")
.setWarningTappedColor("#ff704d")
.setWarningBoxPosition(Noty.WarningPos.BOTTOM)
.setAnimation(Noty.RevealAnim.FADE_IN, Noty.DismissAnim.BACK_TO_BOTTOM, 400,400)
.show();
We changed the default background color, tapped color, warning position and we gave an animation to it. Noty provides you 4 types of animations: Slide in, Slide out, Fade in and Fade out. You can do an animating warnings and change their animation durations also.
But what if we want to know when the user clicked on the warning or the action button? Noty also provides us handy callbacks. For the tap event, there is a tapListener. For clicking the action button, there is clickListener. Here are examples for them below.
Noty.init(YourActivity.this, "Your warning message", yourLayout,
Noty.WarningStyle.SIMPLE)
.setTapListener(new Noty.TapListener() {
@Override
public void onTap(Noty warning) {
// do something...
}
}).show();
Noty.init(YourActivity.this, "Your warning message", yourLayout,
Noty.WarningStyle.ACTION)
.setClickListener(new Noty.ClickListener() {
@Override
public void onClick(Noty warning) {
// do something...
}
}).show();
Note that you can also use all listeners at once.
The last thing I should mention is animation listeners. Noty is providing us animation listeners too. We may want to know the time when the animation started or stopped. The all listeners Noty providing are given below.
Noty.init(YourActivity.this, "Your warning message", yourLayout,
Noty.WarningStyle.ACTION)
.setAnimationListener(new Noty.AnimListener() {
@Override
public void onRevealStart(Noty warning) {
// Start of reveal animation
}
@Override
public void onRevealEnd(Noty warning) {
// End of reveal animation
}
@Override
public void onDismissStart(Noty warning) {
// Start of dismiss animation
}
@Override
public void onDismissEnd(Noty warning) {
// End of dismiss animation
}
}).show();
You can use animation listeners with the tap and click listeners at once too.
Conclusion
If you want to create colorful and animated warnings easly instead of boring toast messages and dialogs, you can try Noty. You will like it.